In Mobile App Development
Calculator App Android Studio Kotlin Tutorial - read the full article about app development tutorial, Mobile App Development and Native and cross-platform solutions from Code With Cal on Qualified.One
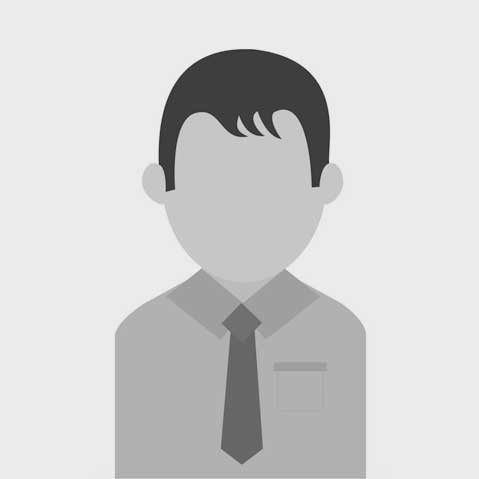
Youtube Blogger

hello and welcome to this kotlin and android studio tutorial my name is cal and in todays tutorial were going to be building a simple calculator were going to be building this calculator from scratch i have made another video where we built a calculator in android studio however in that video we used a third-party plug-in to do all the hard work of the math for us today our calculator is a little bit simpler but were going to be the ones responsible for doing all of the math in the first part of the tutorial were just going to get the layout sorted so were just going to be using some styles and some linear layouts and then in the second part of the tutorial were going to link up all the buttons and make them work this is just really the bare bones of a calculator but i could imagine in the future creating more videos where we build upon add different functions and that sort of thing but yeah hopefully by the end of this tutorial youll have a functioning calculator which you can then build upon with all that said lets get started also creating a new android studio project im going to call this one kotlin calculator app our programming language is kotlin and im just going to hit finish the first thing were going to do is head into values themes and just delete the night theme this is just to simplify it a little bit were going to remove all of the default colors and add in a few of our own so im just creating a red color a very dark gray color that im calling almost black as well as an orange so thats just a pretty simple calculator color scheme and then were going to head into our themes were going to remove all of the secondary brand color stuff all of the comments and just changing our color primary to be orange and our color primary variant to be our almost black and then were going to change the parent theme to app compat and we can close off both of these now and head into our main activity firstly were going to change the constraint layout to a linear layout setting the orientation to vertical background were going to give it our almost black color then inside our linear layout were going to create a constraint layout im going to give it width match parent height 0dp and a weight of 2. were going to give a little bit of padding so padding 20dp and then inside here im going to copy and paste in our textview so this is going to be our workings textview as well as our results textview so im just going to copy and paste that down so weve got two text views the first one im going to call workings text view and the second one were going to give it an id of results text view were just going to change the width to match parent on both were going to give the top one constraint bottom to top of and giving it our results text view were going to set the max lines and lines to 2 on our workings text view as well as setting the lines and max lines to 1 on our results were going to set the text color to be white as well as the text alignment to be text end were going to make the text size of our results 40sp so quite big and just making our workings 25. cool so thats all looking pretty good so im just going to remove the hello world text from both of those text views and then below our constraint layout were going to add in a linear layout giving it a width of match parent height of 0dp and a weight of 1. so this linear layout is going to be each of our button rows and were going to copy and paste this quite a few times so what im going to do is create a style so ive just created a new values resource file calling it styles.xml right click and split vertically to have both xml files on the screen at once inside here were going to create a style im going to name it button row and our button row style is going to have the same width height and weight as the linear layout we just created and then that way we can then apply that style which is our button row to our linear layout and then when we copy and paste this linear layout and then say we want to change something in the future theres only one place we need to modify that styles is one of those things that you can overuse and under use so if you just use a style for absolutely everything it actually becomes more of a hassle to maintain likewise if you never use them and you just copy and pasting over and over again you know thats harder to maintain as well cool but with that said we are going to create a button inside our linear layout and were going to create a style for this button because were going to have a lot of calculator buttons with similar attributes so im just going to copy and paste our button row and rename it to button number and then apply that style to our button im going to give our button a text of 9 and then im going to change the width to 0dp as well as the height to match parent and correctly apply the style to our button just so its calling at style and then giving a button number for our button number style were going to give it a background of null im going to give it text size of 25sp and then im going to copy and paste that button four more times because thats how many columns there are in a calculator changing the text to seven eight nine and then its going to be our time symbol were going to set the text color to white of our button number and then were going to copy and paste our button number style and renaming it to button operator and so our button operator is going to have a different on click and a different text color so our button operator im going to apply that to our time symbol and change the text color to orange i find the easiest way to create an on click for a button that youre going to put in a style is to just create in the button first with error message just create the function inside our main activity so then we can add that on click to our style just calling it operation action and the other one is our number action and then we can remove the on click from those buttons because obviously theyre going to inherit that from the style cool so we can close off our styles.xml just to give ourselves a little bit more space and then were just going to quickly add this ignores hard-coded text to our parent linear layout and then im going to copy and paste down our button row with all the buttons included copy and paste that down four more times and then just go through and change all of the text to being the nice calculated grid for the equal sign were just going to remove one of the buttons so im going to make the weight equal to two a little bit later on for our top left were going to create an all clear button so im just going to change the text color to red so were overriding the style there as well as the on click were going to create an all clear action and then were going to do the same thing were going to create a backspace button and were going to call that backspace action clicking on the error message to create that function in our main activity kotlin file and then just change all of our operators to being divide minus and then addition for our equals button were going to set the weight to two were going to set the background color to orange were going to make the text size 40sp and then were going to override our on click were going to create an equals action in our main activity cool so thats all of our layout sorted next were going to head into our build gradle and add the kotlin android extension and then were finally ready to head into our main activity kotlin and lets start with one of the easier ones first so im going to start with our all clear action and because we added the kotlin extension to our build grader we can now reference text views directly workings text view and results text view are the ids that we gave our text views and im just going to set their text equal to an empty string for our backspace action we just want to remove the last character from our workings text view im going to create a variable calling it length which is equal to workings textview length if length is greater than zero so theres actually something to backspace workings textview text is equal to workings textview text subsequence starting at zero and ending at length minus one so next were going to work on our number and operation action im going to add a variable calling it can add operation which is equal to false which is going to do a little bit of validation for us and then its going to open up the brackets of both of those functions and then inside our number action if view is of type button were just going to say workings text view dot append so adding the text from whichever button is pressed so for example a nine or a seven im going to put that into our workings textview and then were going to copy and paste that down into our operation action but were also going to say if we can add an operation and by operation i mean a times or a plus symbol so when we add up an operation we want to set can add operation to false and then when we add a number we want to add can add operation to true and the other thing we want to validate for is a decimal place so im just going to create another variable calling it can add decimal and thats going to default to true we only want a digit to be able to have one decimal place when operation is added were just going to say can add decimal is equal to true and then inside our number actually were going to say if view text is equal to a decimal place were going to say if you can add a decimal add that to our workings text view and set can add decimal to false otherwise were just going to append whatever digit is given cool so lets build and run this really quick and just see that we can add a digit we can use our backspace button as well as our all clear button you probably cant tell but ive been pressing the operator buttons over and over again and you can only add one operator at a time as well you can only add one decimal place to any given set of numbers so yeah im pretty happy with how thats turned out now its just time to work on our equals action so inside our equals action im just going to say results textview.txt and create a function calling it calculate results which returns a string and im just going to return an empty string for now and then were going to create another function were going to call it digits operators and it returns a mutable list of any type so it could be any type of object inside here were going to create a list calling it mutable list of any and were just going to return that list im going to create a variable calling it current digit which is equal to an empty string were going to say for character in our workings textview.text so go through all of our workings and if you find a character which is a digit or you find a character which is a decimal place current digit plus equal to that character otherwise youll find an operator which means were going to add our current digit to float so were going to make a float out of that current digit string that weve made were going to set current digit back to an empty string and then were going to add that character which is adding the operator and then outside of our loop were just going to say if our current digit is not equal to an empty string were going to add that digit to float to our list the idea of this function is to break up our workings text view into an array with floats and characters as operators cool so inside our calculate results were just going to put that list into a variable and then were just going to say if our digits operator is empty were just going to return an empty string next were going to work out the times and division as BODMAS says you need to do division and multiplication before you do any add or subtracting so im just going to create a new function calling it times division calculate which takes our digits and operators list and returns a mutable list of any were going to create a variable called list which is equal to pass list and were going to say while our list contains a times or a divide symbol were going to create another function which returns a mutable list of any this function is going to do one times or division at a time so just going through the list and doing one at a time and then returning that list were going to create another variable calling it new list which is a mutable list of type any were going to return our new list and then were going to create a variable calling it restart index which is equal to our past list size then were going to create a for loop to go through our pass list so im just going to say i in past list indices if pass list at position i is a character so meaning its an operator and i is not equal to past list last index meaning its not the last index and i is less than our restart index were going to say value operator is equal to our past list at i previous digit is going to be past lists it should actually be i minus 1 as float and our next digit should be past list plus 1 as float so weve got our operator and weve got the two digits we want to times or divide and then we want to say when our operator is a time symbol we want to say new list add previous digit times our next digit and we just want to do the same thing as a divide symbol but just changing that to division previous digit divided by next digit and then inside both of them were going to say restart index is equal to i plus 1. otherwise youve found a addition or a subtraction were just going to say new list add previous digit were going to add our operator and then below that but still inside our for loop were going to say if i is greater than our restart index new list at the object from our pass list at the index of i cool so that should be our times and division all sorted so im just going to copy and paste this if digits is empty mine and just change that to our times and division because were just going to return an empty string if that is empty the final thing left to do is create a function which calculates our add and subtraction this function is going to receive a mutable list of any but its going to return a float so ill just put that into a variable inside here were going to say result is equal to past list at the index of zero take the first float variable and put it into our result variable just going to return our result were going to say for i in past list indices so just looping through our past list again we say if our past list at index of i is a character meaning its an operator and i is not the last index of our past list so very similar to our validation in times and division were going to say our operator is equal to our past list at position i our next digit is equal to our past list i plus one as float im going to say if our operator is equal to an addition result plus equal to our next digit and then were going to copy and paste that down and same thing for negative so just minus equals to next digit just going to change this to result dot to string and we actually need to fix up a bug here when i didnt add i plus 1 or i minus 1 in our times and division so just changing that now and if we go and run this now you can see that we can add and subtract we can also do a simple times as well well test the division and it should be able to add multiple divisions or multiple times or plus or whatever you want really and it should result in the correct calculation yeah i hope you enjoyed building this kotlin calculator from scratch i hope you learned something if you made it this far consider giving the video a like or subscribing otherwise ill catch you in the next tutorial
Code With Cal: Calculator App Android Studio Kotlin Tutorial - Mobile App Development