In Software Development
Learn C++ With Me #18 - Vectors - read the full article about C++ 2021, Software Development and from Tech With Tim on Qualified.One
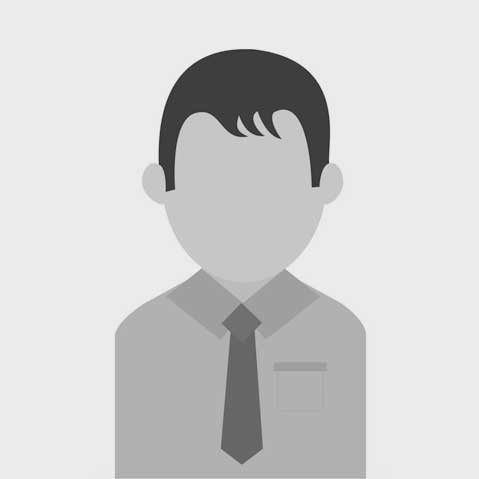
Youtube Blogger

hello everybody and welcome to another c plus plus tutorial for beginners in todays video ill be covering vectors now a vector is a dynamically resizable array this means that the vector can change its size so unlike an array that is a set static size that cannot change if you try to add more elements into a vector and it is not large enough it will just increase itself now theres a lot of complexities involved with that but anyways well talk about them in this video all right so the first thing were going to do here is include vector so were going to say include if i can spell include correctly not happening very well here we will include vector and just a note here if youre ever noticing that for some reason im using some data type or object thats not working for you make sure you have the included statement right so previously i think we used maps and you needed to include that we had use some other things you need to include as well and sometimes like stuffs kind of works but then doesnt work and anyways just always check the include statement because thats probably like a pretty easy fix to a lot of problems you guys might be running into anyways what is a vector as i was saying a vector really is just like an array except it has a lot of extra functionality so it is kind of wrapping an array and allowing this array to dynamically change its size and when i say dynamically change its size as i was kind of stating in the introduction this means that we dont need to know how big the vector is when we first create it i dont need to define that its only going to have five elements in or 20 elements or whatever i can do that theres options to specify how big the vector actually is but i dont have to and that also means that if i decide to add something into the vector its not large enough it will just expand itself i can also have the vector shrink itself so that any of the kind of extra space its allocating for new elements it no longer is using in our computers memory anyways its best if i just show you a few examples so lets say vector were going to define the type of the vector which in this case is going to be int and then we need the name so in this case say v1 and this is going to be equal to and then just like an array so 1 2 comma 3. so now ive created a vector you can see there are no problems here and well that is how you make a vector so obviously you need to pick one type you put it in the angle brackets then everything in the vector must be that type so theres all kinds of stuff we can do with a vector first thing we can do is we can look at the position of different elements right lets say v1 at index 1 we can end l here thats going to give us 2 because well thats index 1 of the vector index 2 will give us 3 and there you go now we can also look at the front of the vector so i think i dont know if i need parentheses or not but i believe i can do front and yeah thats gonna give me one and then i think i can do back and that is going to give me the end so to get the start and the end of the vector you can do the front and the back now i have a little cheat sheet on my uh kind of right monitor here because theres so many different ones to go through those are kind of the main ones in terms of accessing elements in the vector so very similar to what you use for an array then we have the size uh this will tell us the number of elements in the vector so three obviously if i change this like four you know now we have four i can also look at whats known as the capacity of the vector now this one im going to do some kind of fancy examples to show you how this changes right now the capacity of this vector is four but if i decide to add some elements to this vector which i will show you how to do youll notice this capacity is not necessarily going to be the same as the size of the vector so the capacity is how many elements it can currently hold and the size is the number of elements its actually holding so if i decide to add an element to the vector and the way i can do this is v1 dot and then push back now what this means is push an element from the back of the vector so this is kind of equivalent to appending an element so adding to the end of the vector is what this really means and i decide to push maybe like nine here lets see if the capacity changes notice that i added one element and my capacity increased not by one it increased actually by what was mapped there by four it doubled the size of the vector so now the capacity is eight so lets actually add a total of eight elements or add a number of elements that we get to eight so if i do this now i push four nines and i push one more then technically our vector should be of size nine right so now if i do this notice that the capacity goes up to sixteen so whats actually happening here is as soon as i add an extra element and lets actually do this here so when we add just eight the capacity stays at eight as soon as i add this ninth element it knows that the vector is not big enough so it doubles the size of the vector right thats kind of the pattern were running into now we get 16. and so if we print out the capacity and then we print out sorry we dont need two semicolons here we print out the size of the vector you see were going to get 16 with the size of 9.
so hopefully thats clear but that is kind of how the vector works its just increasing the size of itself as required and its trying to do it by an amount where its not going to have to constantly increase the size because increasing the size takes a long time to do theres a few more things that we can do with vectors here so i was pushing from the back but i also can pop from the back or i believe push from the front im trying to see if i have that uh theres nothing for push for the front but ill show you how we can like add something to the front of the vector um lets also see here again just kind of scanning my cheat sheet okay ill show you pop from back so if i pop from back what im doing is removing an element from the back of the vector now when i say pop from back i dont need to give any index or anything and what this will do is return to me the element that was removed and it will remove the very last element from the vector but youll notice what happens here im going to add four elements in and im going to pop four elements off and im going to print the size and the capacity at kind of each step here so after we add all of the elements on im going to look at the capacity and the size im then going to pop off four elements and then well look at the capacity and the size of the vector so lets do this and notice that we get 8 8 and then we get 8 4. and so the capacity of the vector did not change even though we removed elements from it and so thats something to keep in mind that even if i say pop one more off of here so lets actually copy this line and do this lets see what we get here we get eight eight eight three so the capacity is not going to decrease when you remove elements its only increasing so if you want to decrease the capacity of a vector and again the reason why you would actually even want to do this is because you dont really want to have a vector that has like one element in it thats using a capacity of say 10 000 or something crazy because in that situation youre just wasting a ton of space your vector is kind of taking up this space in memory and saying okay i have this much room and its using that space and so if you dont need to be using that space you shouldnt so if you remove a ton of elements from a vector what you can do is shrink that vector and the method to do this is shrink to underscore to underscore fit uh let me make sure thats the correct one i think thats right uh i want to make sure were not doing this wrong yes uh shrink to fit that is correct so if we run this now uh oops we need a semicolon so lets add that notice that what we get now is 8833 and so it shrank the vector to fit the number of elements that were in it so the capacity of the vector is equal to its size after we say shrink to fit and so those are kind of the core methods for the vector now the few uh the next things ill show you sorry is to insert and erase elements from the vector so popping from back was pretty straightforward we were removing an element from the end of the vector and so we would have removed these uh four nines and then i guess actually the four after we removed all of that so now how do we insert and kind of erase specific elements so lets erase all of this so we will continue in one second but i need to quickly thank the sponsor of this video and the series which is algo expert algo expert is the best platform to use when preparing for your software engineering coding interviews they have over 150 coding interview questions on the platform which are taught by the best instructors and curated such that you are not wasting your time going through a ton of problems that you will never be asked in a coding interview anyways check out algo expert from the link in the description and use the discount code tech with tim for a discount on the platform okay so the next thing im going to show you is how to insert and erase elements now this is not necessarily super intuitive to actually be able to insert an element you need a pointer to the position of the element that you want to insert this element before and so lets kind of look at this here what i can say is v1 dot insert and then if i wanted to insert at the very beginning i would say v1 dot begin and then i would say what i want to insert which is 5 and now if i see out v1 at index 0 lets just end l here and i run this notice that we get 5.
so we inserted at the very beginning right the pointer to the kind of first element here of the element 5. now if we do this again and we do 7 this will now insert before 5. so now notice that we get 7 printing out there now if we say v1 dot begin and maybe we add 1 to this well lets just see whats going to happen now and yeah lets see what we get when we print out v1 at index 0.
notice that we get 1 but if we print out v1 at index 1 we are getting 5 the element that we inserted at position one so before the element at position one which means that this element will be at whatever position we define here and so thats kind of how this works now this is not really the best way to go about doing this like v1.begin plus one im actually i havent tested if what im about to do is gonna work but lets try this lets try v1 at position and lets go with position maybe yeah we can actually we can do zero and lets see if that actually works here i dont think thats going to work yet no that doesnt work but if i try an ampersand here and do that yeah okay so it still doesnt work so we need to do a dot begin and then we can kind of add to dot begin to figure out where we actually want to insert this element now unfortunately i dont really like how this works i wish that there was a way that we could do it just based on the index maybe there is a way to do it just based on the index if there is let me know please in the comments but regardless im going to show you now how we can erase so to erase is a very similar thing what were going to do is say b1 dot arrays and then obviously we dont need to like put an element if were going to erase one we just need to put what element we want to erase so in this case well say v1 dot begin and then this will erase the element thats at this position to the very first position so if i do this notice that we now get two printing out because we well we erased one okay so thats kind of all the core methods and functions i want to show you relating to the vectors the next thing im going to show you is how we iterate through a vector so im going to get rid of this erase here and im just going to show you how we can do this theres a few different ways the first way is to do something like into i is equal to 0.
i is less than v1.size notice im using the size not the capacity thats very important plus plus i and then i do something like co to be v1 at index i and then end l okay so if we run this we get one two three four as we would expect now thats fine we can go with that method or what we can do is say auto itr is equal to v1 dot begin we can say itr does not equal v1 dot end and then plus plus itr and lets see what we get here if we say c out and we see out itr now lets give a test here lets run this notice were getting an error no match for operator blah blah lets see what happens now if we do an asterisk so if we do an asterisk notice how now were actually getting the proper elements because we had to de-reference this pointer so this actually works all right so thats another way you can do this again this gives us all the elements now lets just see what happens if i decide to insert an element in this vector so i say maybe after we do the first one v1 dot insert lets insert at the beginning so v1 dot begin lets insert 10. now lets run this with the iterator we need a semicolon okay now notice we get 10 1 2 3 4 and so all is working all is fine so yeah thats how you iterate through a vector and really theres not much more for me to show you there is a lot of other methods and stuff involved with a vector that you can use with a vector these are kind of the core ones again this is what you would use when you need a kind of dynamically sized structure that can increase or decrease its size just be careful when youre using a vector because if you remove a ton of elements from a vector you could be in a situation where well you have just a ton of space being used by this vector and well it only really needs to soar like two or three elements so anyways with that said i hope you guys enjoyed if you did make sure to leave a like subscribe to the channel i will see you in another youtube video
Tech With Tim: Learn C++ With Me #18 - Vectors - Software Development