In Mobile App Development
Tic Tac Toe Game Swift Xcode Tutorial | Noughts & Crosses - read the full article about app development tutorial, Mobile App Development and Native and cross-platform solutions from Code With Cal on Qualified.One
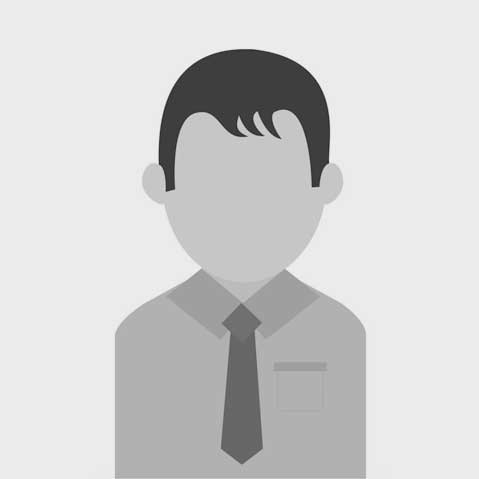
Youtube Blogger
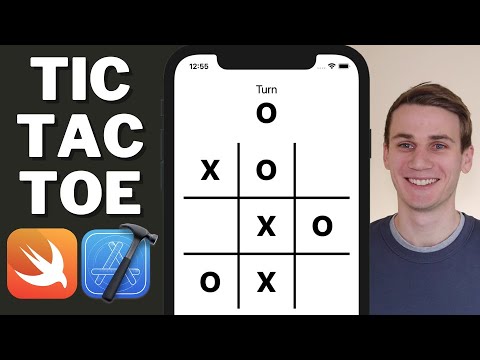
hello and welcome to this swift and xcode tutorial my name is cal and in todays tutorial were going to be building a tic tac toe game or noughts and crosses this is far and away an original idea when it comes to a idea for a beginner programming project but i think what makes it such a great beginner programming project is that we all know how it should look we all know what the end result should be but there are just so many different ways that you can go about solving this problem so this is just one solution of how to create a tic-tac-toe game using swift and xcode hopefully we learned something and lets get started starting off were just going to create a new swift and xcode project product name is tic-tac-toe or noughts and crosses i think if youre from the uk interface storyboard programming language is swift heading into the main storyboard were going to create our 3x3 grid so im just going to drag in a vertical stack view im going to set the background color to black set the distribution to fill equally and then im going to right click and just say aspect ratio and then were also going to set it horizontally and vertically in the safe area as well as giving it equal widths now were going to change our proportional width to 0.9 and were going to set our aspect ratio to one to one so its a square so its the same width and height and then inside our vertical stack view were going to add in a horizontal stack view were going to set distribution to fill equally on our horizontal stack view as well as well as giving it some spacing and we actually need to give our vertical stack view the same spacing so were going to give it five and five so thats how thick our black lines are going to be and then were going to drag in a button just making sure that it lands inside our horizontal stack view so you can see the nesting on this left pane here cool so next we want to set our buttons background to white so im just going to scroll down and set the background to white were going to make our text color black as well as making the font size 60 and the font style to be heavy and then were going to give our button a placeholder so just a cross and then im just going to click on our button and copy and paste that two more times and then before we copy and paste down our horizontal stack view im just going to remove the titles and then were going to copy and paste down our stack view two more times and there you have it you have a nice 3x3 grid and obviously the vertical stack view has the black color but then with the white buttons over the top created a scaling grid that doesnt require like a background image or anything like that so this should work on any screen size so the final thing were going to add to our layout is a turn label so im just going to drag in a label and give it top space and center horizontally in the safe area im going to make the font size a little bigger as well as changing the text to term and then im going to copy and paste that label and then just set it just below our turn label im going to make the text a lot bigger so im going to make it heavy and 60 so to match our buttons and then im just going to give it a cross cool and now were just going to create some outlets so im just going to open up the assistant editor right click and drag from the cross above you did load im going to give the outlet a name of turn label and then were going to right click and drag from each one of our buttons giving the first one a1 and the second one a2 and then our next row is going to be b1 b2 and the final row would be c1 c2 and c3 and then were going to right click and drag below our view did load and create an action and our sender is of type ui button and then were just going to drag from our board tap action were just going to drag that onto all the buttons so no matter which button is pressed were going to run this function you can double check that all of the connections have been made correctly by hovering over this dot and seeing that they all light up on the storyboard cool so thats it for our layout we can close off this system editor now and head into our view controller swift file first thing im going to do is declare an enum were just going to call it turn with a case of nought and cross theres absolutely no reason why you couldnt use a boolean or an into or really anything to determine whose turn it is but i found that using this enum here is the easiest way to do it and sort of the most reader-friendly solution cool so next what were going to do is just declare a couple of variables the first one were going to call first turn which were going to initialize to turn cross and this is just going to keep track of whose turn it is to go first in each game and then were going to create another variable also with turn cross and thats the current turn thats going to flip-flop each time a tile is placed and the first turn is going to flip-flopping every time a game ends cool so just below that were going to declare a couple of constant strings so just calling the first one nought and the next one cross so this is just literally the character that were going to place so weve got a o and a capital x and now below our board tap action were going to create a function calling it add to board which receives sender so the specific button in our grid thats been tapped and were going to say if our sender.title for state normal is equal to nil so meaning theres nothing in our buttons title inside that statement were going to say if our current turn is equal to turn nought were going to set the title to our nought for our state normal were going to set current turn equal to turn cross and our turn label text to cross and then we basically just need to do the opposite so were going to say else if our turn is our crosss turn were going to set the title to cross and set current turn and our turn label both to nought and now we just need to call from our board tap action add to board giving it the sender button cool so if we build and run this now we can see that you can add a nought or a cross and the turn label is updating correctly you cant override a nought with a cross or a cross with a nought cool so next were going to deal with a draw so we just want once the board is full we want to be able to reset the game before we do that though at the bottom of our add to board were just going to set sender enabled as equal to false thats just to remove the animation when you click a button that already has a nought or a cross placed and then im going to create a variable calling it board which is an array of buttons its going to create a function calling it init board and in here were just going to say board append a1 and were just going to do that for every single one of our buttons so a1 a2 a3 so on and so forth cool so just below our board tap action were going to create a new function which were going to call full board and it is going to return a boolean and all this function is going to do is check to see if theres an empty space on our board so were just going to say for button in board if the button title for state normal is equal to nil meaning that you found an empty space return false otherwise were going to return true and then from our board tab action were going to say if the board is full so if full board is equal to true were going to create another function were going to call it result alert which is going to take a title string and then inside here were going to say let ac equal to ui alert controller just giving it a title of title message is nil and our preferred style is action sheet were going to say ac add action giving it a new ui alert action the title here were going to give it reset and the style is default and then were just going to hit enter and create a handler were going to create a function calling it reset board which were going to call from our handler so were just going to say self reset board and then below that were just going to present our alert controller by calling self.present giving it our alert controller and animated is true cool so our reset board function should be fairly simple we just want to set all of the buttons on our tic tac toe board back to nil so just looping through each of our buttons so full button in board button.set title giving the title nil for our state normal and then we just want to set enable back to true so button is enabled is equal to true so regardless of the result of the game we want the first turn variable to flip-flop if the first turn is equal to our noughts turn first turn is equal to turn cross and our turn label.text is equal to cross and then were just going to copy and paste down that function changing it to an else if statement and doing exactly the opposite so if the turn is the turn cross first turn is equal to turn nought and our turn label.txt is a nought and then were just going to say current turn is equal to first turn cool and now we need to just start this chain off so if board is full we just want to return our result alert giving it a title of draw cool so lets check in to see if thats all working so im just going to keep placing noughts and crosses until theres no more spaces and we see that alert there and we hit the reset button and the board sets back to empty and then well do it again and just keeping an eye out for the turn label and it seems to be all working correctly the final thing we need to do now is create a function calling it check for victory were going to call this function before our full board function just to check to see if someones won tic-tac-toe meaning that theyve got three in a row so im just going to create a function calling it check for victory which receives a string and returns a boolean and return false for now create another function below that which is called this symbol which is going to take a ui button as well as a string calling it symbol also going to return a boolean in this function is just going to return if the button title for state normal is equal to the symbol so if we pass in across does the button title have a cross and if so return true so now before we return false we just want to check for all the different victory conditions so were just going to start horizontally so were going to say if this symbol a1 and this symbol a2 and this symbol a3 meaning that theres either a nought or a cross on the top row if this is the case were going to return true and then we just want to do that for each of the horizontal victories so were going to do b1 b2 and b3 as well as c1 c2 and c3 and then we just want to copy and paste all three of these checks and were going to check for a vertical victory so a1 b1 and c1 a2 b2 and c2 as well as a3 b3 and c3 and then we just need to check for one more victory type which is the diagonal victories so theres actually only two of these so weve got a1 b2 and c3 as well as a3 b2 and c1 and now before our board tap action we can say if check for victory giving it across so were going to check to see if the cross has had a victory and if so were going to return our result alert with the crosses win title and then below that were going to check to see if the nought had won and just changing the title and well quickly implement a little scorecard thing so im just going to create another variable calling it nought score equal to zero and then below that crosses score is also equal to zero and then were just going to say crosses score plus equal to one we actually need to do that before our results alert and then for our nought victory were going to say nought score plus equal to one and then in our result alert were going to create a little message here just giving an update on the score so just saying noughts plus the string of our nought score and crosses plus the string of our crosses score and then we just need to feed that message into our alert controllers message and lets build run this one last time and just check to see if we can get a victory and there you have it thats a very simple tic tac toe game in swift and xcode or noughts and crosses game a simple tic tac toe game really is a fantastic beginner programming project because theres so many different ways you can solve the same problem i really had a lot of fun building this one and ill catch you guys in the next tutorial
Code With Cal: Tic Tac Toe Game Swift Xcode Tutorial | Noughts & Crosses - Mobile App Development