In Mobile App Development
10 minutes to build a game in Flutter - read the full article about flutter app development, Mobile App Development and Native and cross-platform solutions from Filip Hráček on Qualified.One
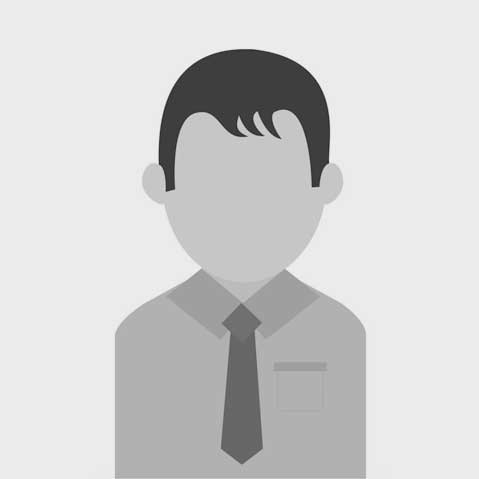
Youtube Blogger
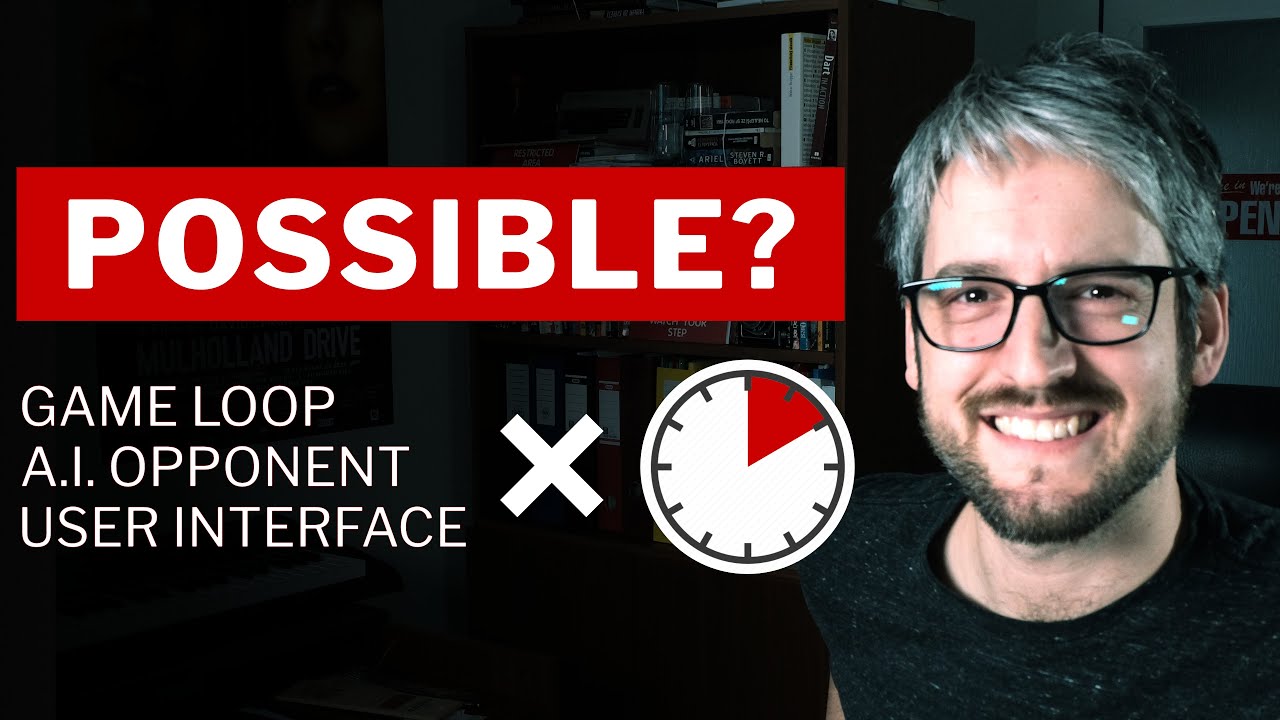
Ever since I left Google, a few months ago, I wanted to do two projects which I couldnt do when I was still employed at Google. I mean, I *could* do them, but I didnt have the balls to do them. Yeah.
The first project is what this whole video is about, and its basically me trying to implement a full game in Flutter in 10 minutes.
And I mean, not 10 minutes of edited video, but 10 minutes straight from start to finish. Without using any editing. Without using any pre-existing code, or anything like that.
Just from the Counter app to a finished game, in 10 minutes.
So, its probably going to fail, but anyway... Ill try it.
So I guess I can start. Let me start the timer. And... go! Hey! This is me from the future. I realized that I couldnt actually talk while also building this game, so instead I deferred to my future self to do the talking and the explaining. And so thats what Im doing here.
All right, so, so far, I just replaced everything under the Scaffold of this app, which is the the usual [Counter] app, with a Row.
And in it, theres an AspectRatio of 1, which just makes sure that this will be a square. And in it, theres a GridView.
So Im still not telling you what this game is about, but it has a grid.
It has a grid of nine fields by three, so 3 by 3. And see, I have trouble actually writing code here. Like, actually writing characters. Which doesnt bode well for the whole experiment.
Okay, so I have something there. I just saved, and therefore there was a hot reload, and so you can see over there, on the left... or *right* bottom corner, that I have some some kind of a grid there.
And next to the AspectRatio, next to the square, I have a Column which is basically my status part of the game. Which, right now, just says "Your move." And then there is a button which, again, I have trouble actually editing stuff, apparently, and it just says "Restart." Right, so it doesnt do anything yet. Also, I dont want everything pressed so hard to the top of the page, so a little bit of nicety is to put the mainAxisAlignment to spaceAround. Which makes a little more room to each item in that list.
And also, putting everything (every tile) into Center.
In this, I will not do a lot of visual stuff, of course. I have only 10 minutes. But still, its nice.
Cool. I have created the state of the game now, which is a list of nine zeroes at the start.
But its going to always be a list of nine numbers.
Now, ideally, if I were doing an actual game, on a grid, I would of course want to have a two-dimensional list, or array, or something.
But in this case, I am realizing, of course, that I dont have the time to do this. And I can easily, basically, hard code everything there.
So, now I can actually check [the game state]. And you can see that Im showing nothing when the the tile says zero, I am showing X when its one, and O when its two. I think you can guess what Im going with.
And now Im allowing the player to actually interact with the game, by adding an InkWell that says tiles[i] = 1.
And now I tried it, and it shows that, yes, it works. The player can actually add Xs on this [grid].
And so, now, were going to, like, program the AI. The AI is basically the the major problem of this game. Because we already have the UI. Its terrible, it looks bad, but its there.
So the AI first needs to wait for some duration, and this is completely just for the feel of it. If you start... if your AI responds too early, it doesnt look like an AI. It looks fake. So you have to... and this is actually true even in normal games (you can trust me, Im a game developer in part). Some games could work much faster but they still give that little amount of time so that it looks more like youre playing against an opponent instead of playing against the computer that could immediately respond.
Cool, so the AI needs to go through the playing field. Now, I think you already know whats going on. Its tic-tac-toe. Its a tic-tac-toe [game] where you can play with the AI. And, tic-tac-toe has a grid of three by three tiles. And you can see that Im just basically hard coding that this tile is zero, one, two, you know, up to eight. And now I just go... the AI just goes through each of the tiles and it constructs a "future", like a possibility in the future, of what the tiles could look like. So this one line, on line 137, is basically, [it] says: copy all the the current state of the world into tiles, into the variable `future`, and also change the tile on tile `i` to 2.
Which means, the opponent, the AIs tile. And if this is winning... we havent implemented `isWinning` yet, but if this is a winning "future", then assign `i` to `winning`. Which means, we know now that this particular number is the winning [tile], and we should probably take it. And also, [we] try to figure out if this... Again, change the "future" to "if this [tile] was taken by the player". And, if it was taken by the player, and the player would win, then assign this to `blocking`. Which means, we should probably block this. [We should block] the player from playing this one.
Cool, all right. So, then we check: is `winning` not null? If not, then thats our move. If yes, if [winning] is null, then try `blocking`. And if not, then try `normal`. And if we have a move to play, then play it.
Cool, so now we need to implement `isWinning`, actually. So, for that, we basically just need to check for eight different possibilities, right? So, the fact that `who` is winning, can be either that, for example... I have a problem here that I will fix in a second.
But if `tiles[0]` is `who` (which is either 1 or 2 — either player or the AI), if its uh... If that is... I mean, if that is `who`...
Basically, if they all belong, all these tiles belong to the player that were asking about, then this is a winning state. And we should return true, right? And now Im basically going, and listing all the possible ways that a player or the AI could win. So the first one is: "we have the whole row". On to the next one, and the next one. And then there is the this diagonal, and then [that]diagonal. And then the [columns], right? So thats the eight different ways, and I have to use my brain now... My past self needs to use his brain to figure out which numbers should go here.
You can see that Im not that bad at it, actually, because I have everything in front of me. Right? So I can kind of see, "oh, zero one two is the front row, and then [go] from there". Its like, the first three rows are kind of a cheat sheet for me.
Cool, so now I have the `isWinning` method. I actually think Im already done. But Im going up, to change the status so that if Im winning, if the player is winning, then it shouldnt say "Your move", but it should say "You won!" If the AI is winning, it should say "You lose!" or "You lost!" or something like that. I dont remember exactly.
And if not, then we say "Your move." Cool, so, that works.
And now Im trying... my past self is trying to restart. And then Im realizing, oh, I havent implemented that yet.
So, now, Im implementing the Restart button. Which, basically, all it does is it changes the tiles back to zero. Everything needs to be zero. And of course this is in `setState`. So, nine zeros.
Cool. Save, and now restart should work. And now I can change things... yeah. And you can see that I was blocked by the AI.
Im basically done now. I dont know it... My past self doesnt know it yet but Im done.
And everything works as I would expect. Im just testing now.
Cool, now Im trying to win, Im trying to find out if I can win. And you can see me losing. I cant win in tic-tac-toe! I mean, I can, of course, but like, I was so worried that Im missing something that I just kept losing.
But I think now I will understand that, yes, okay, this is how you win in tic-tac-toe.
Cool, and thats it. I think I finished before 10 minutes. I just stopped it, at 10:29:48, but Im pretty sure I was finished before.
So I call it a success.
Cool, so, yeah.
Wow! That...
Im pretty happy. I mean, the code, of course, is terrible.
Just terrible. But, you know, what do you expect for 10 minutes, right? Wow.
All right. And it works. It has a restart button, it even wins. The AI, you know. So, pretty cool.
If youre interested in some of the shortcuts that I used while I was working on this, you should check out my course. I just published a course on Udemy about Android Studio shortcuts. So you could check it out.
And if you want to know what the other project is, Ill tell you. Its...
The other project that I didnt have the balls to do when I was at Google is trying to build...
a full operating system in Flutter.
I know, yes. But, its less ridiculous than you think.
Anyway, have fun, and see you around!
Filip Hráček: 10 minutes to build a game in Flutter - Mobile App Development