In Game Development
How do you evaluate the performance of a gaming computer? Let's see 6 ways of FPS calculation
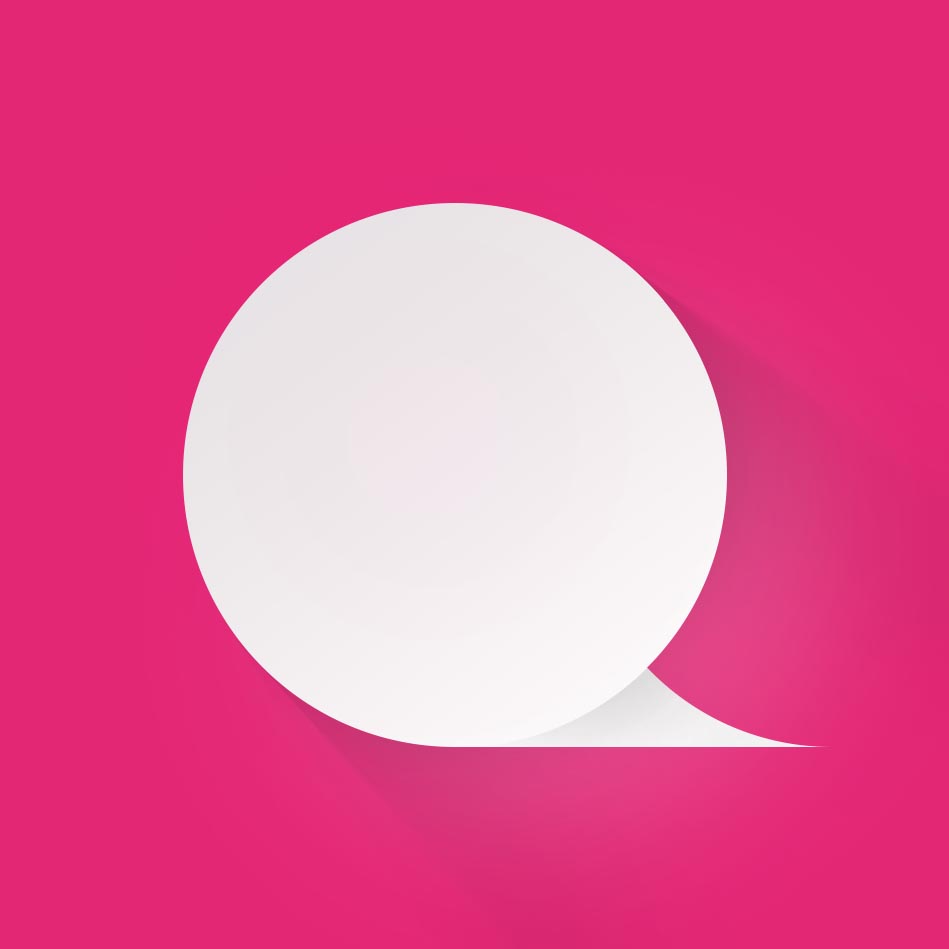
Management
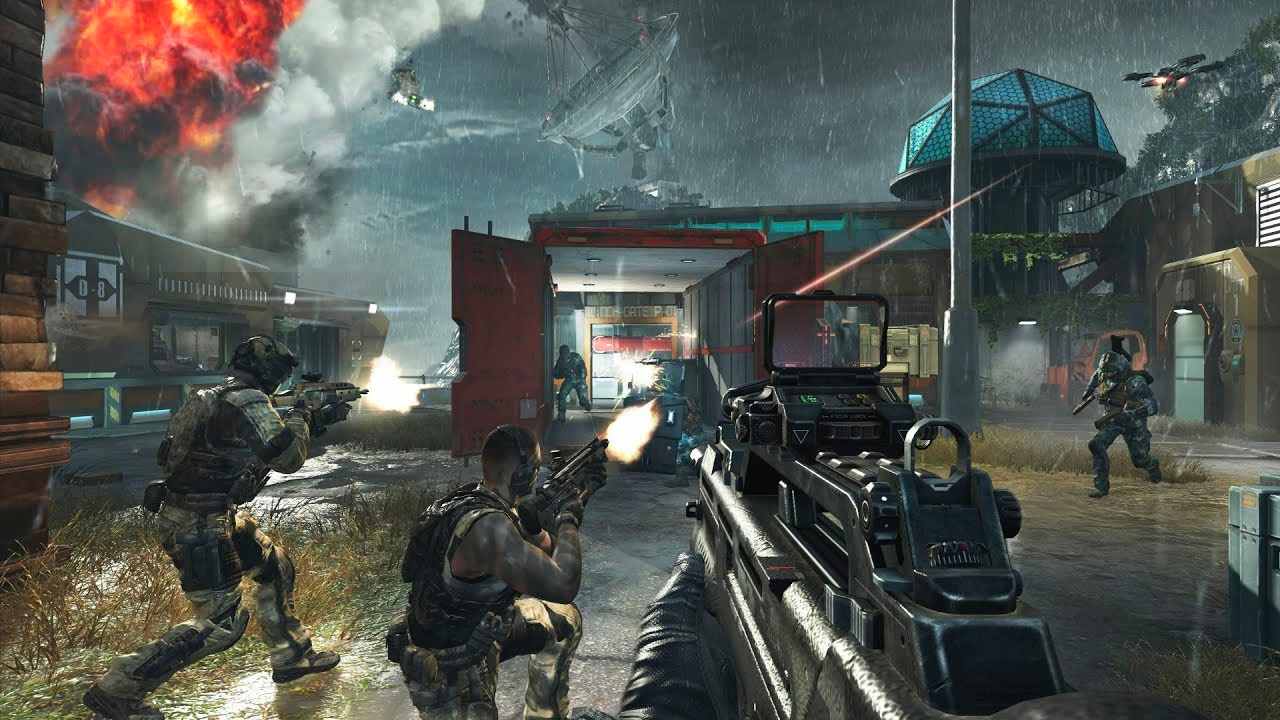
A computer may have the fastest processor capable of performing billions of operations per second, RAM running at exorbitant speeds, and a graphics card supporting all the latest technologies, but only by keeping all these components working together can a truly smooth and immersive gaming experience be achieved. That's why the most important metric for PC gaming performance is Frames per Second (FPS) in today's games.
However, each game requires a different amount of resources to render a single frame, and therefore the number of frames the computer is able to display per unit time will be different for each game. In addition, FPS is significantly affected by factors such as screen resolution (the higher it is, the more work for the graphics processor) and graphics settings in the game (rendering photorealistic effects in the latest games is very resource-intensive).
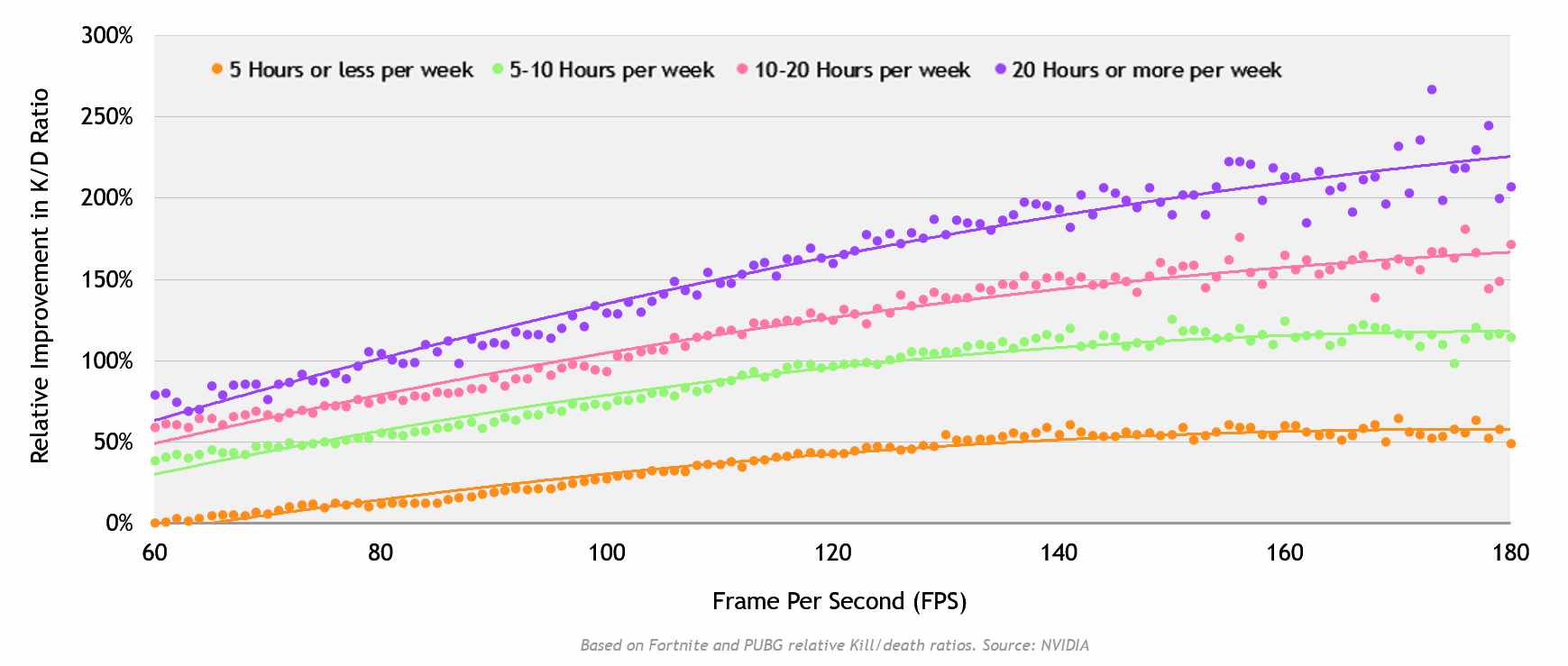
Thus, picking up the necessary requirements, you can estimate the level of performance of the new computer for today's games, and most importantly, track how the gaming performance varies depending on the configuration of the PC.
Calculating frame rate (FPS) is a common topic in game programming. In general, there are six ways.
Fixed interval method of FPS calculation
A formula for calculating frame rate:
fps = frameNum / elapsedTime;
If the number of frames for a fixed time is recorded, you can calculate the frame rate. This method is used more frequently.
int fps()
{
static int fps = 0;
static int lastTime = getTime(); // ms
static int frameCount = 0;
++frameCount;
int curTime = getTime();
if (curTime-lastTime> 1000)
{
fps = frameCount;
frameCount = 0;
lastTime = curTime;
}
return fps;
}
There is another method of writing:
int fps(int deltaTime)
{
static int fps = 0;
static int timeLeft = 1000; // Fixed interval = 1 second
static int frameCount = 0;
++frameCount;
timeLeft -= deltaTime;
if (timeLeft < 0)
{
fps = frameCount;
frameCount = 0;
timeLeft = 1000;
}
return fps;
}
Fixed frame time method of FPS calculation
The formula for calculating the frame rate:
fps = frameNum / elapsedTime;
Frame rate can also be obtained by calculating the time used by the number of frames for each fixed number of frames. This method is used less frequently.
int fps()
{
static int fps = 0;
static int frameCount = 0;
static int lastTime = getTime(); // ms
++frameCount;
if (frameCount> = 100)
{
int curTime = getTime();
fps = frameCount / (curTime - lastTime) * 1000;
lastTime = curTime;
frameCount = 0;
}
return fps;
}
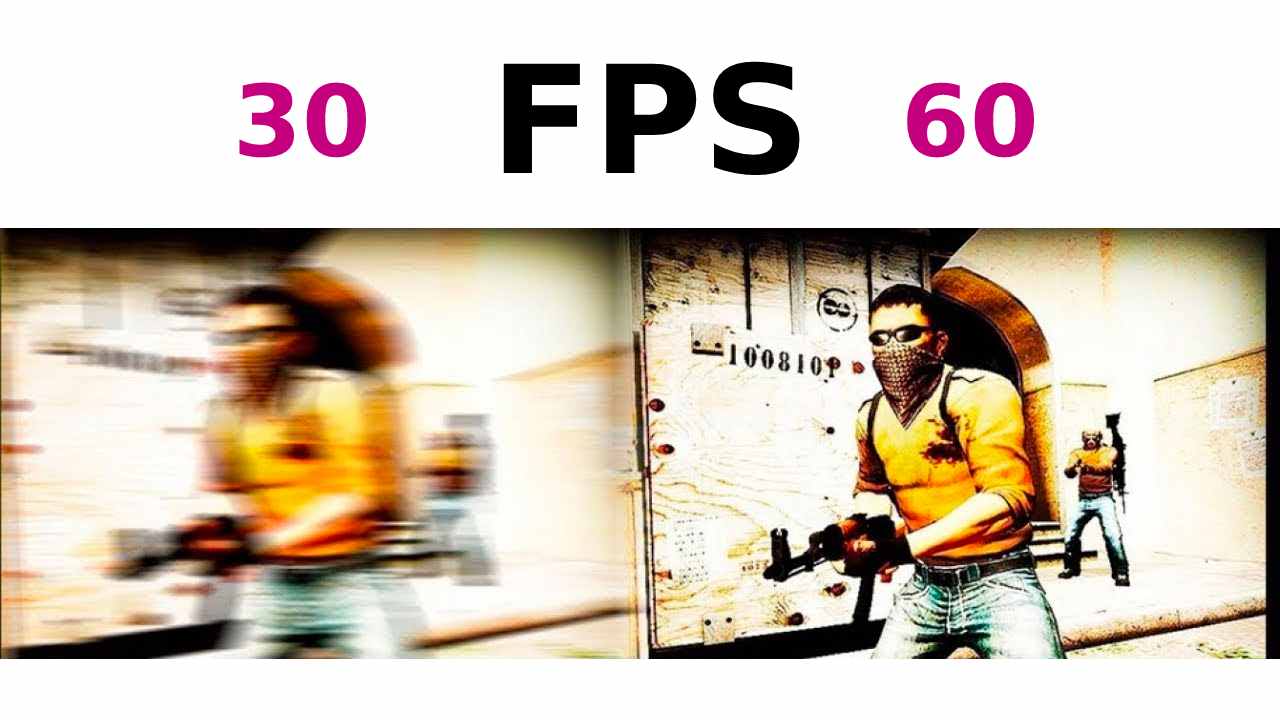
Real-time FPS calculation method
The real-time FPS calculation method directly uses the time interval of the previous frame to calculate.
int fps(int deltaTime) // ms
{
int fps = static_cast (1.f / deltaTime * 1000);
return fps;
}
Common average method
The general average FPS calculation method uses global frame number divided by global time to find frame rate.
int beginTime = getTime();
int fps()
{
static int frameCount = 0;
++frameCount;
int deltaTime = getTime() - beginTime();
return static_cast (frameCount * 1.f / deltaTime * 1000);
}
Exact sampling method
The exact sampling FPS calculation method samples the first N frames and then calculates the average. This method requires additional memory space, so it is not normally used.
int fps(int deltaTime) // ms
{
static std::queue q;
static int sumDuration = 0; // ms
int fps = 0;
if (q.size () <100) // Количество сэмплов установлено на 100
{
sumDuration += deltaTime;
q.push(deltaTime);
fps = static_cast (q.size () * 1.f / sumDuration * 1000.f);
}
else
{
sumDuration -= q.front();
sumDuration += deltaTime;
sumDuration.pop();
sumDuration.push(deltaTime);
fps = static_cast (100.f / sumDuration * 1000.f); // Не забудьте преобразовать в число с плавающей запятой, иначе точность будет потеряна
}
return fps;
}
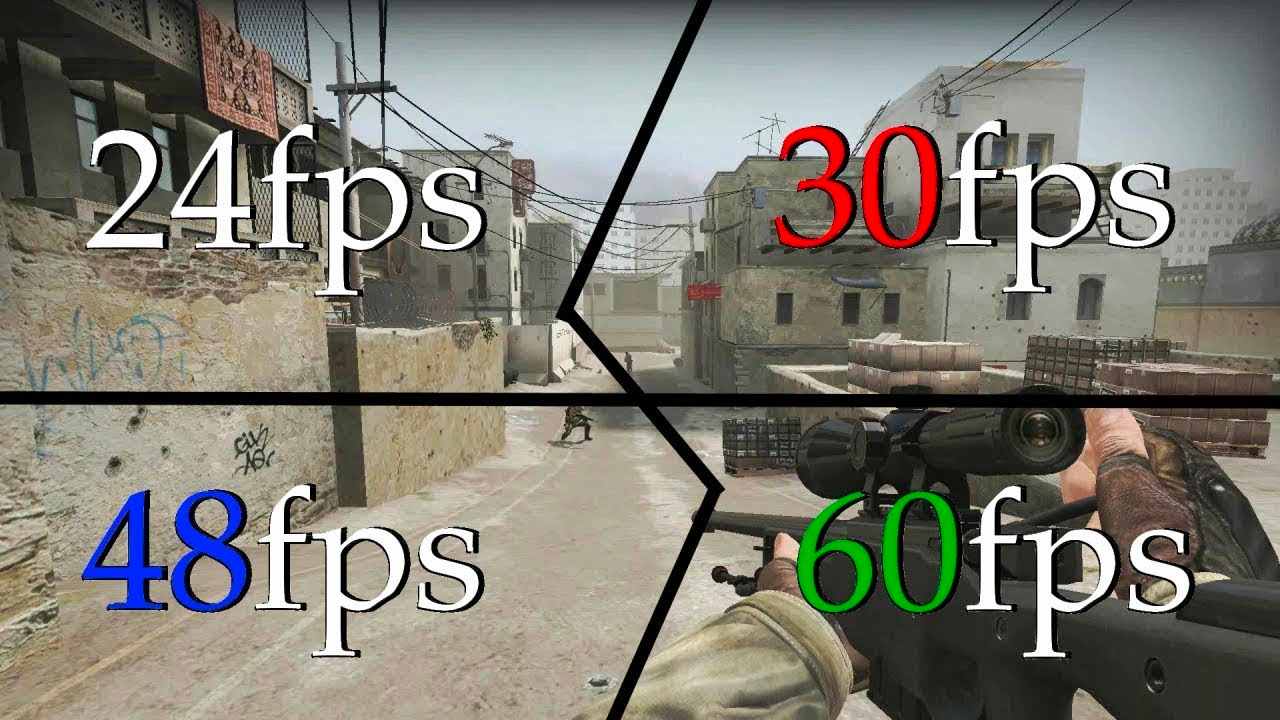
Average sampling method
The average sampling FPS calculation method uses previous statistical results, overcoming the disadvantage that the exact sampling method requires additional space. This method is used more frequently.
int fps(int deltaTime) // ms
{
static float avgDuration = 0.f;
static alpha = 1.f / 100.f; // 100 samples
static int frameCount = 0;
++frameCount;
int fps = 0;
if (1 == frameCount)
{
avgDuration = static_cast(deltaTime);
}
else
{
avgDuration = avgDuration * (1 - alpha) + deltaTime * alpha;
}
fps = static_cast(1.f / avgDuration * 1000);
return fps;
}
Gaming PC FPS Comparison
Looking for cheap PCs for Apex Legends, Red Dead Redemption, Fortnite, Minecraft or even Sims? Click on the options below, narrow down your choices and use our charts to find the best deal for PCs.
The best PC gaming allows you to enjoy the latest AAA games the way they were meant to be played, whether you're copying and ripping in Doom Eternal or silly scaring yourself in Resident Evil 3.
And why wait for the PS5 and Xbox Series X? Once you've chosen the right gaming PC, you can take advantage of features like ray tracing, 4K and 60fps gaming, and fast SSD loading right now. Building a PC is a great option for those who want to customise things exactly the way they see fit, but there are plenty of great pre-built gaming PC options that provide great performance at a reasonable price.
But while gaming PCs are a great way to enjoy the latest games with incredible settings with minimal hassle, you have a lot to learn before you spend thousands of dollars apiece. And with so many models to choose from, which include PCs from leading manufacturers, finding the right PC for your budget can be a challenge.
That's where we're at. We regularly research, test and analyse the most popular gaming desktops to help you find the best gaming PC for your needs.
Buying the best gaming PC in terms of FPS
Buying the best gaming PC as a pre-built system can be the best place to start when you're passionate about PC gaming or when you're hitting a wall trying to upgrade your current system. Sure, building your own setup can be extremely satisfying, but simply buying a complete gaming PC will take all the stress out of a home project and give you something to turn to if, god forbid, something goes wrong.
While part of the innate beauty of a gaming PC is the ability to upgrade components to improve performance, this capacity is limited. There is a tipping point when further upgrades become unprofitable and a rebuild becomes necessary. And this is where buying the best gaming PC you can afford makes a lot of sense.
Getting a good deal on a pre-built gaming PC will take just as much research as building a great high-end PC build. Some system builders will try to saddle you with a kit that charges extra for things you might not need, such as overclocking, RGB, a monitor or keyboard and mouse. Even if you do need it, there's often a good chance of getting something better for less money.
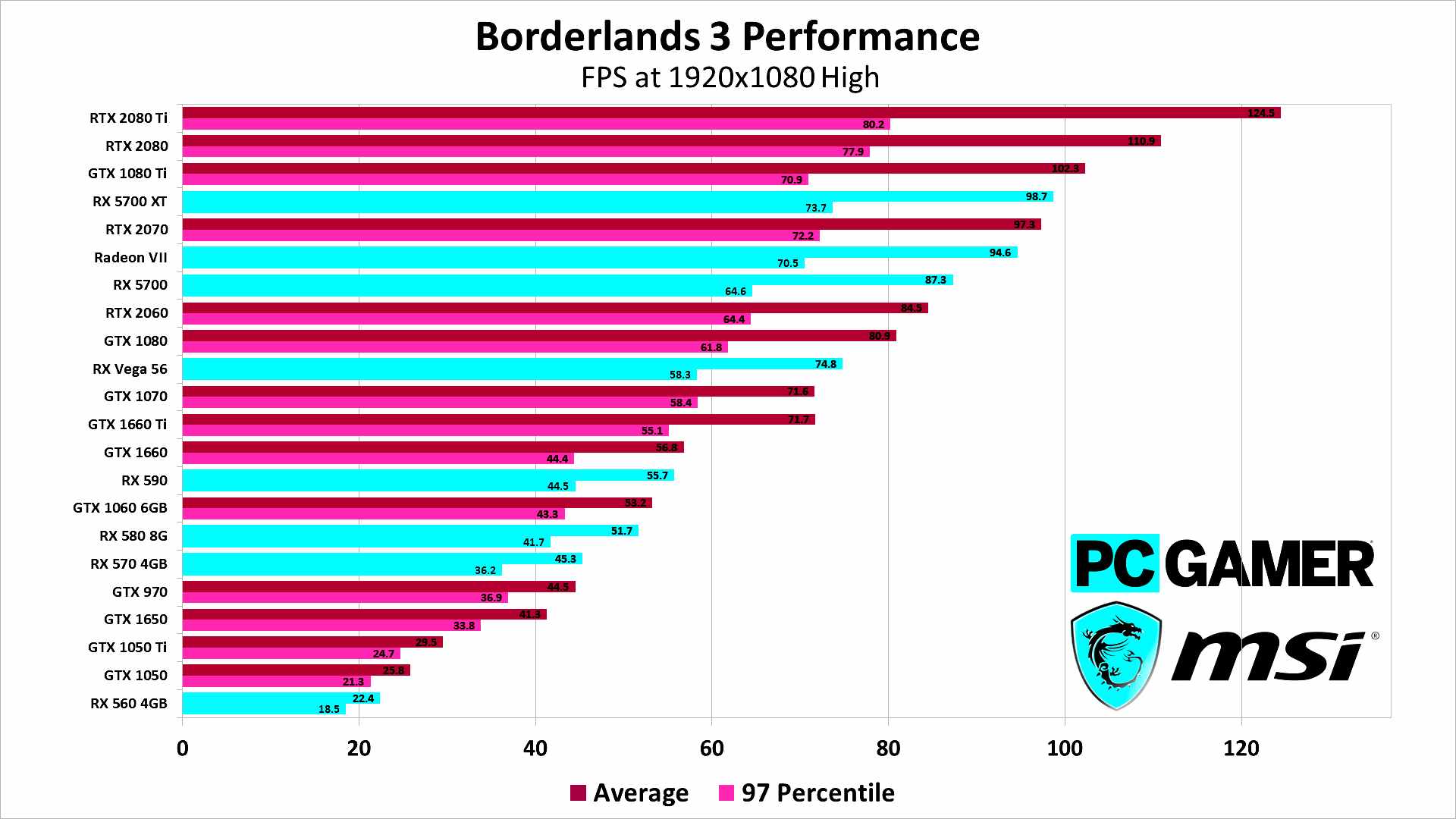
Our top priority is balancing price and performance. Ideally, your gaming PC should have one of the best graphics cards and the best processor for gaming, although this is not always financially possible. But you can't skimp on one and overdo the other; the Nvidia RTX 2080 Ti is only worth having in combination with a high-end processor that can harness its power. Then there's support. Aftermarket support is where a good system builder becomes a great system builder, and this is where it might make sense to buy a ready-made machine.
All experienced gamers wonder about building a gaming PC at some point and whether it's worth it. It's a great option if choice and do-it-yourself don't scare you - sometimes it's the only way to get exactly the configuration you want - or if you think it'll be fun. But as a rule, it's not the way to save money compared to an identical ready-to-ship model.
It can be cheaper than getting a special premium model from companies like Origin PC, Falcon Northwest, Digital Storm and so on. There are few things more frustrating than preparing and sitting down to play the last AAA only to have it fly out during the opening cutscene and only have yourself to blame.
The other high-end solution you might encounter is a desktop or laptop, especially since 17-inch gaming laptops with desktop-class processors and GPUs, such as the Alienware Area-51m and Acer Predator Helios 700, offer desktop-level performance with the convenience of a similar all-in-one. All-in-one with a really fast, gaming-optimised display. While these larger laptops usually support upgrades, it's usually not as cheap or easy to do as with even the cheapest desktop computer.
Choosing the best PC for gaming is always a compromise. Every game uses system resources - processor (also known as CPU), graphics processor (GPU), memory (RAM), storage - differently and often horribly inefficiently. You can't even expect resource usage to be the same for a particular genre of game, such as a first-person shooter (FPS), platformer or simulator, because optimization levels can vary widely. Gaming computers (and content creation computers) are the unhappy babies of consumer electronics: they're loud, wayward and require constant supervision. And just when you think you've got them under control, they roll into crazy town.
FPS calculation: Intel vs AMD processors
Unless you buy a custom build or do it yourself, you really can't pick comparable configurations to mix and match. Manufacturers tend to choose configurations based on what they think will be popular at a given price level. Choose your preferred graphics card and then see what CPU options are offered within your budget. AMD tend to have lower clock speeds - they have higher base frequencies and lower acceleration clock speeds - but better multi-core performance for the same money. If your favourite games are older, they probably don't use more than four cores (if that) and will probably give you the power you need from Intel's fast individual cores. However, AMD's latest processors have significantly narrowed the single-core performance gap with Intel, and almost all of them support overclocking (only the Intel K-series does).
Unless you have unlimited funds, the price tag will also play a part in your decision. If you're looking for a decent computer with basic to medium specs, you can expect to pay around $699. If you're willing to spend around $1,000, you're likely to find a solid tower with medium to high specs. And if you're feeling good and are willing to shell out $1,500 or more, you can buy a very high-end system with top-notch features such as multiple GPUs and at least two drives (hard or solid state).